Shortcodes are a powerful feature in WordPress that allow developers and site owners to add dynamic content in posts, pages, and widgets without writing complex code. They are particularly useful for embedding functionalities such as buttons, forms, and galleries with minimal effort.
When creating custom shortcodes in WordPress, you often need to pass parameters to customize their behavior. These parameters, known as “shortcode attributes,” enable flexibility and better control over the output. Understanding how to retrieve and use shortcode attributes properly ensures your WordPress development is both efficient and scalable.
What Are Shortcode Attributes?
Shortcode attributes are key-value pairs that modify the behavior of a shortcode. They are included within the shortcode tag inside square brackets. For example:
[custom_shortcode title="Hello World" color="blue"]
In this example, the shortcode has two attributes: title and color. These attributes can be accessed using PHP in your WordPress plugin or theme files.
How to Retrieve Shortcode Attributes in WordPress
To retrieve attributes in a shortcode, WordPress provides the shortcode_atts()
function, which helps handle default values and user-defined attributes safely.
Step 1: Define the Shortcode
First, you need to register a new shortcode using the add_shortcode()
function in your theme’s functions.php
file or a custom plugin.
function custom_shortcode_function($atts) {
// Define default values
$defaults = array(
'title' => 'Default Title',
'color' => 'black'
);
// Merge user inputs with defaults
$attributes = shortcode_atts($defaults, $atts);
// Retrieve attribute values
$title = esc_html($attributes['title']);
$color = esc_attr($attributes['color']);
return "<h2 style='color: $color;'>$title</h2>";
}
add_shortcode('custom_shortcode', 'custom_shortcode_function');
Step 2: Use the Shortcode in Posts or Pages
Now, you can insert the shortcode in your WordPress editor as follows:
[custom_shortcode title="Welcome to My Site" color="green"]
The function will take the user-defined attributes and merge them with the defaults, preventing errors when values are missing.
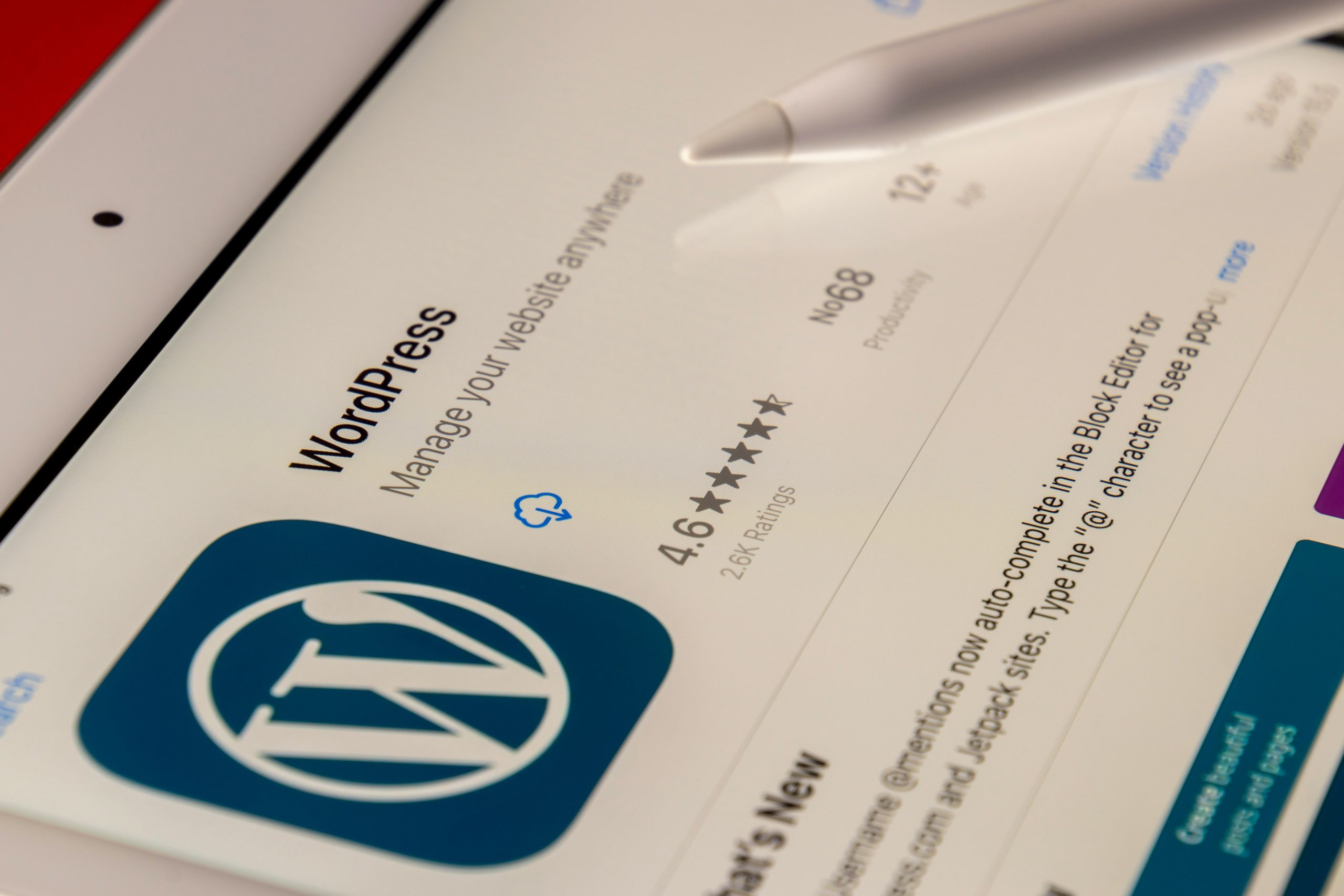
Handling Missing or Unexpected Attributes
When handling shortcode attributes, it’s important to consider cases where users may provide invalid or missing values. The shortcode_atts()
function ensures:
- Default values are used when attributes are not provided.
- Unexpected attributes do not break functionality.
- User inputs are sanitized to prevent security vulnerabilities.
For instance, wrapping the output in functions like esc_html()
and esc_attr()
ensures that the attributes are safely rendered in HTML.
Using Boolean Attributes
Sometimes, you may want to use attributes as flags that enable or disable certain features. Boolean attributes work best when values are explicitly set:
function custom_boolean_shortcode($atts) {
$defaults = array(
'enable' => 'false'
);
$attributes = shortcode_atts($defaults, $atts);
$enabled = filter_var($attributes['enable'], FILTER_VALIDATE_BOOLEAN);
return $enabled ? "<p>Feature Enabled</p>" : "<p>Feature Disabled</p>";
}
add_shortcode('boolean_shortcode', 'custom_boolean_shortcode');
Now, using [boolean_shortcode enable="true"]
will return “Feature Enabled,” whereas omitting the attribute or setting it to “false” will return “Feature Disabled.”
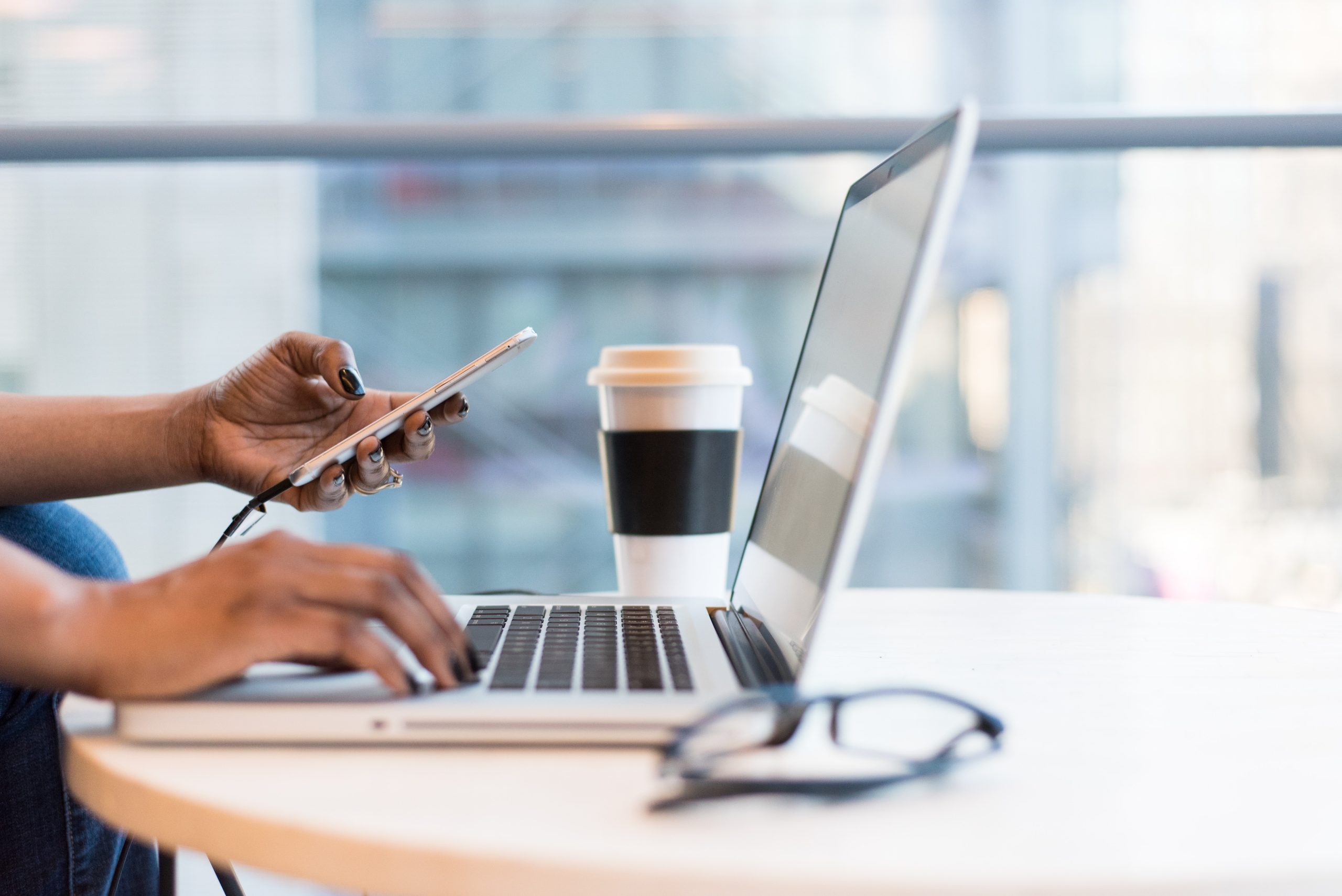
Using Nested Shortcodes
WordPress allows shortcodes to be nested within one another. However, for a shortcode to properly process nested content, you need to modify the shortcode function:
function nested_shortcode($atts, $content = null) {
return "<div class='nested-content'>" . do_shortcode($content) . "</div>";
}
add_shortcode('nested_shortcode', 'nested_shortcode');
Now, if a user places another shortcode within [nested_shortcode]
, it will be processed correctly.
Common Mistakes to Avoid
When working with shortcode attributes, developers often make common errors. Here are a few things to watch out for:
- Not defining
shortcode_atts()
, which may lead to undefined index errors. - Failing to sanitize user input, which can create security vulnerabilities.
- Omitting
do_shortcode()
when handling nested shortcodes. - Using incorrect data types, such as setting boolean attributes without checking their value properly.
Conclusion
Retrieving shortcode attributes in WordPress is an essential skill for any developer looking to enhance their website’s functionality. Using shortcode_atts()
, sanitizing inputs, and handling edge cases improve the reliability and security of your code. By following best practices, you can create clean, efficient, and dynamic shortcodes that enhance the user experience across different WordPress installations.
Whether you’re building a custom plugin or tweaking a theme, mastering WordPress shortcodes and attributes will help you develop flexible and scalable solutions that fit your needs.