Getting ready for your first .NET interview can feel a bit scary. But don’t worry! With some basic knowledge and practice, you’ll do great. In this guide, you’ll learn about essential .NET concepts in a simple and fun way. We’ll also look at common questions you might be asked and how to answer them.
What is .NET?
.NET is a framework created by Microsoft. It lets developers build all kinds of apps—desktop, web, mobile, and more. You can write code in languages like C#, VB.NET, and F#.
The most popular one is C#. So most .NET interviews will focus on that.
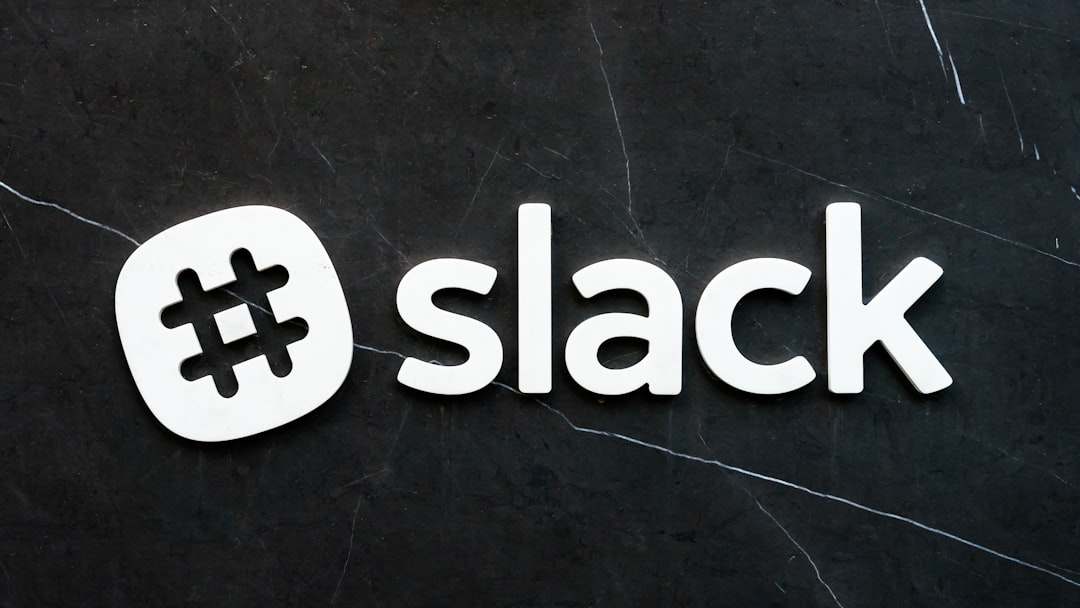
Common .NET Interview Questions (and Simple Answers!)
1. What is the CLR?
CLR stands for Common Language Runtime. It’s like the heart of .NET. It runs your code and manages memory, security, and more.
Interview Tip: Think of the CLR as a helpful robot that takes care of difficult stuff for you!
2. What’s the difference between a Class and an Object?
- Class: A blueprint or recipe.
- Object: The actual thing made from the blueprint.
For example, a class could be Car. An object is myRedCar.
3. What is the difference between Value Types and Reference Types?
This one shows up a lot in interviews!
- Value Types store data directly. Examples: int, char, bool.
- Reference Types store a reference to the data. Examples: class, string, array.
So if you change a value inside a reference type, it can affect the original value!
4. What is a Namespace?
Think of a namespace as a folder. It helps organize your code. Inside the folder are classes, interfaces, and more.
It helps prevent naming conflicts. You can even create your own namespaces!
5. What are Constructors?
Constructors are special methods used to create objects of a class. They set up the class when you make a new object.
public class Dog
{
public Dog()
{
// This is a constructor
}
}
Cool, right? When you say new Dog()
, the constructor runs automatically.
6. What does “Static” mean?
A static thing belongs to the class, not the object.
Example: A static method can be called without making an object.
public static void SayHello()
{
Console.WriteLine("Hello!");
}
7. What are the Main Pillars of OOP?
OOP stands for Object-Oriented Programming. .NET loves OOP! There are 4 main pillars:
- Encapsulation: Keeping data safe inside a class.
- Inheritance: One class can use features from another.
- Polymorphism: One function, many forms.
- Abstraction: Hiding complex stuff, showing only what’s needed.
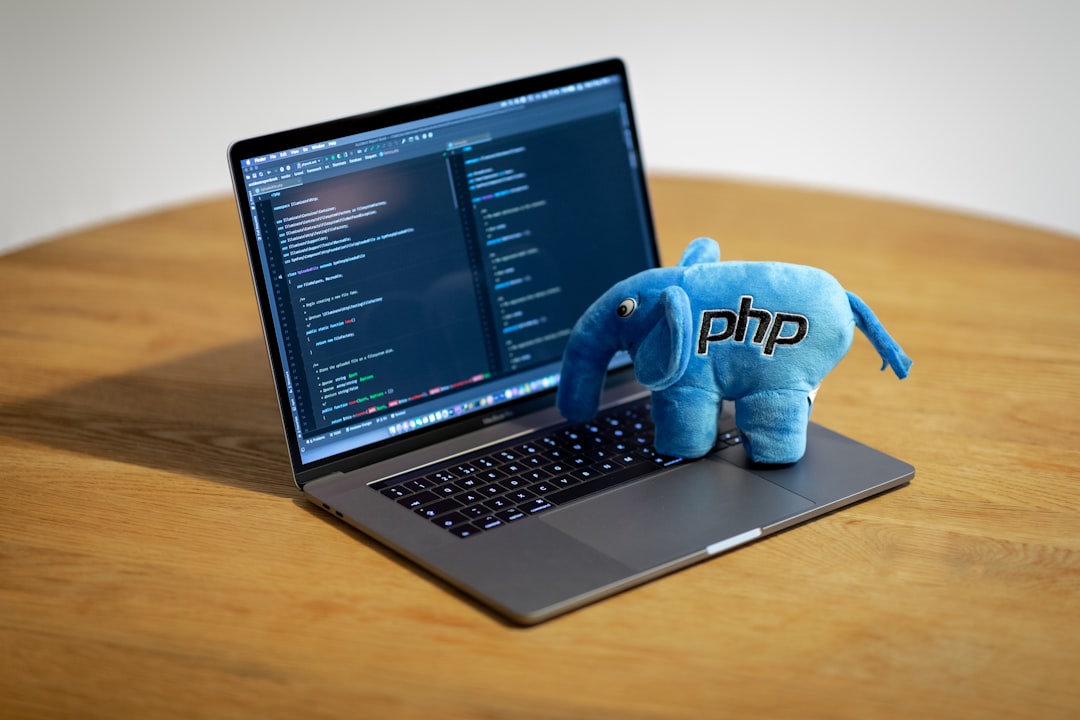
8. What is an Interface?
An interface defines what a class should do—but not how to do it.
Think of it as a contract. If a class signs it, it promises to do the things listed in the interface.
9. What are Assemblies?
An Assembly in .NET is like a package of your code. It usually ends with .exe or .dll. It includes the compiled code and other information needed to run it.
10. What’s the difference between Managed and Unmanaged Code?
- Managed Code: Runs inside the CLR. Safe and easier to control.
- Unmanaged Code: Doesn’t run inside CLR. Examples: C or C++ code.
.NET handles most stuff with managed code. That’s why it’s great for beginners!
Bonus Tips for Interview Day!
- Practice short and simple answers.
- Don’t just memorize—try explaining the concepts to a friend.
- If you don’t know an answer, say so politely. Honesty is better than guessing.
- Smile and be confident. You got this!
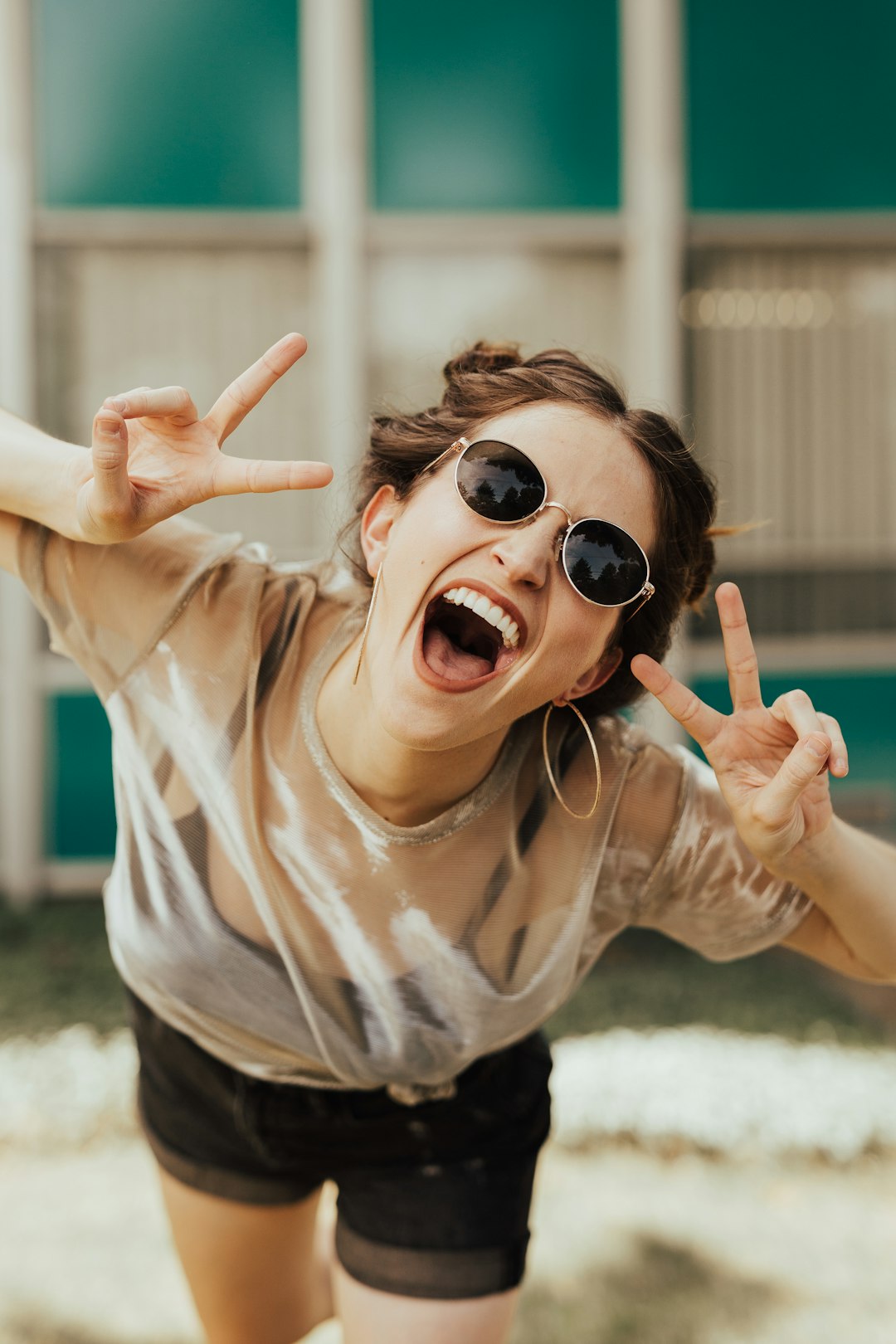
Wrap-Up
The .NET world is huge and exciting. As a beginner, you don’t need to know it all. Just focus on the basics like classes, OOP, CLR, and data types.
Keep learning, keep coding, and soon you’ll be a .NET whiz. Good luck with your interview!