Globally Unique Identifiers, or GUIDs, are a staple in modern software development. These 128-bit values are designed to be unique across time and space, making them ideal for identifying data and objects across distributed systems, databases, and applications. Implementing GUIDs correctly can help developers avoid many issues related to data collisions, security, and performance degradation.
This article will explore the best practices for implementing GUIDs in software development, covering aspects such as generation methods, storage considerations, performance optimization, and tooling choices.
Understanding How GUIDs Work
A typical GUID looks like this: f47ac10b-58cc-4372-a567-0e02b2c3d479
. This format follows the variant and version-based structure defined in RFC 4122. There are several versions of GUIDs, with Version 1 (timestamp-based) and Version 4 (random-based) being the most commonly used. Choosing the right version depends on the use case and system architecture.
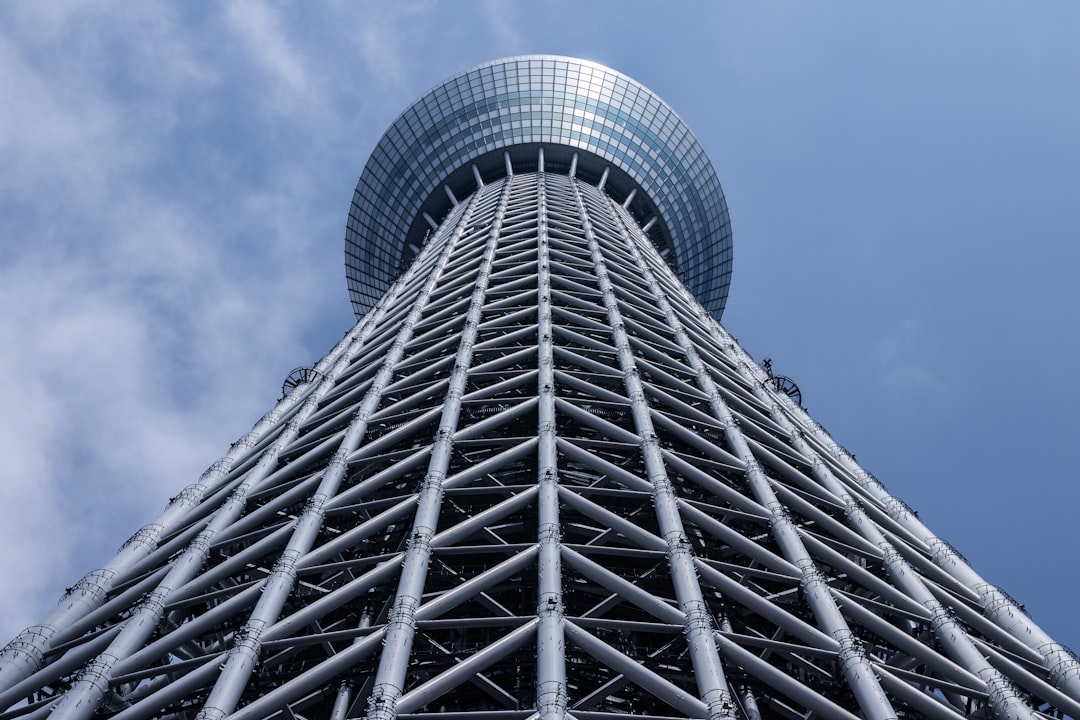
Best Practices for Implementing GUIDs
1. Choose the Right GUID Version
Different projects may benefit from different types of GUIDs:
- Version 1 (Time-Based): Useful when preserving the chronological order of records is important. However, it can potentially expose information like the MAC address of the originating server.
- Version 4 (Random-Based): Offers better anonymity and is suitable for scenarios where data ordering is irrelevant but uniqueness is crucial.
Tip: Be cautious with Version 1 GUIDs in environments where privacy is a priority.
2. Avoid Using GUIDs as Primary Keys in Relational Databases
Using GUIDs as primary keys in SQL databases can lead to performance issues due to their non-sequential nature. Large index fragmentation and slower query performance are common side effects.
Instead, consider:
- Using sequential GUIDs when supported by your database (e.g., SQL Server’s
NEWSEQUENTIALID()
). - Using integer-based IDs for internal operations and GUIDs only for external references.
3. Store GUIDs Efficiently
A GUID is typically 16 bytes (128 bits), but when logged or displayed, it often appears as a 36-character string. When storing GUIDs in databases:
- Use the
UUID
orGUID
field type if supported (e.g., PostgreSQL, MySQL 8+), as it optimizes storage and indexing. - Avoid storing GUIDs as text where possible to save space and ensure better performance.
4. Ensure Secure Generation
Random number generators are essential for creating Version 4 GUIDs. Always rely on cryptographically secure random number generators provided by your language or platform.
For instance:
- In .NET, use
Guid.NewGuid()
- In Python, use
uuid.uuid4()
- In JavaScript, use libraries like
uuid
withuuid.v4()
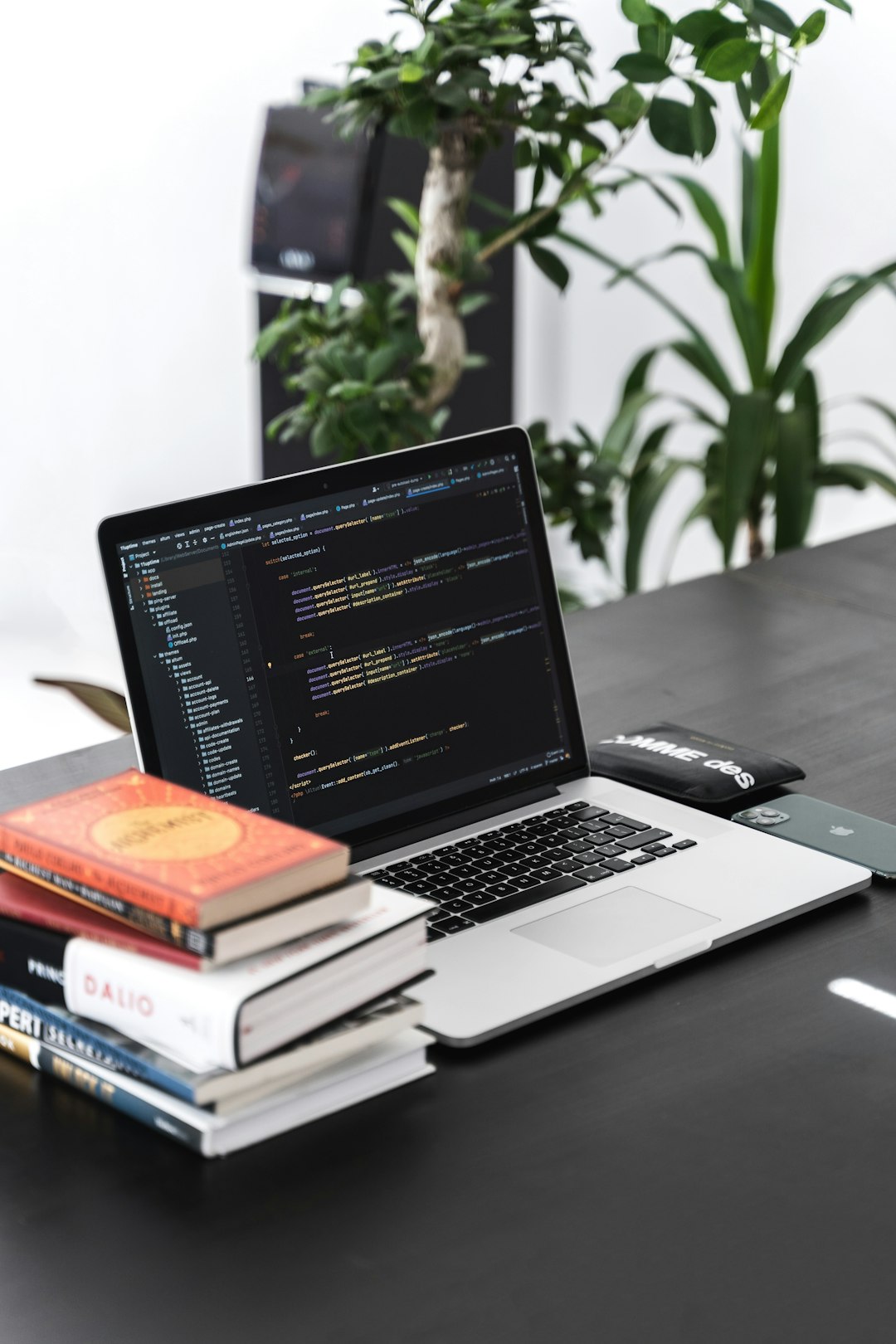
5. Use GUIDs Sparingly in Logs and URLs
While GUIDs are meant to be publicly shareable, adding them to logs or URLs can introduce clutter and complicate debugging. More importantly, it may create unintentional security risks if sensitive identifiers are exposed.
Instead:
- Mask or hash GUIDs before displaying them to end users.
- Use URL-safe formats if GUIDs must be shared over the web (e.g., Base64-encoded GUIDs).
6. Maintain Consistency Across Systems
GUID formats and generation methods can differ across languages and platforms. Ensure consistency by using centralized libraries or APIs for GUID generation in your microservices, especially if services are written in different languages.
Conclusion
GUIDs are an incredibly powerful tool for ensuring uniqueness across distributed systems, but they must be implemented thoughtfully. By choosing the right version, storing them efficiently, securing their generation, and minimizing their exposure, developers can harness the full potential of GUIDs without incurring unnecessary overhead or complexity.
As with any tool, understanding its inner workings and best practices will lead to more robust, scalable, and secure software systems.